Create RNG (Random) dice in Unity
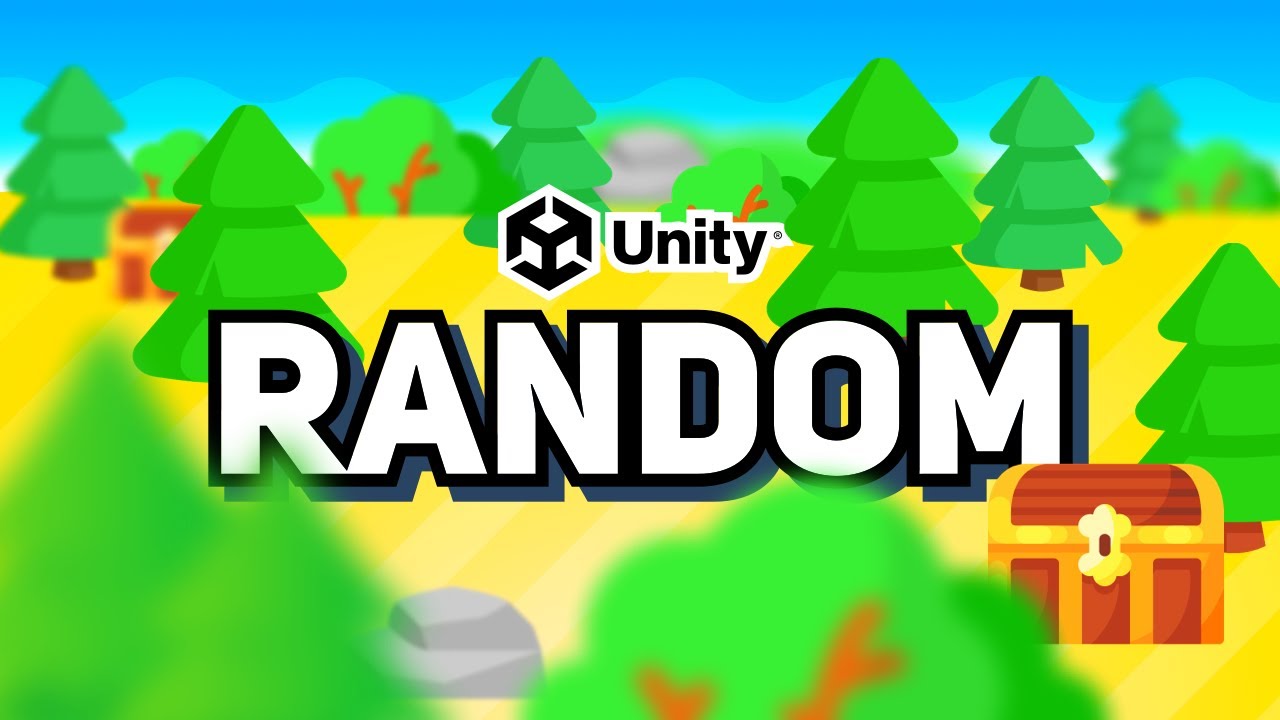
Loading...
RNG (Random Number Generation) mechanic can be both very exciting and frustrating in games. why not add that crazy mechanic to your own projects?
Today, we're going to learn how to create a dice that displays random numbers between one and six.
Let's start by downloading the dice project template for free using the link below:
Setting up dice roll mechanic
- Download above file and open
SampleScene1
- Select the dice sprite in the scene
- In the inspector, click „Add Component”
- Type „RandomGenerator” and select it to create new script
Create the roll method
-
Let’s create a roll method that for now will only say „Rolled the dice” in our console (using Debug.Log):C#
1public class RandomGenerator : MonoBehaviour { 2 3 public void Roll() { 4 Debug.Log("Rolled the dice"); 5 } 6}
-
Save the script, go back to Unity
-
Connect this method to a button (Randomize button), by adding new OnClick Event, dragging Dice to it and selecting RandomGenerator -> Roll()Preview
-
Now press PLAY in Unity and click "Roll" button on the scene., when you press the button, a A message saying „Rolled the dice” will show up in the consolePreview
Add a functionality to the dice
Next, let’s add functionality to update the dice sprite to match the rolled numer.
-
Inside your class add a sprite array as a public propertyC#
public Sprite[] faces;
-
In Unity, fill this array with the faces of your dice in the inspector panel. You can find them in the Assets folder in the provided project.
-
Update the Roll metod to pick a random value and update the sprite:C#
1public void Roll() { 2 Debug.Log("Rolled the dice"); 3 int faceIndex = Random.Range(0, faces.Length); 4 GetComponent<SpriteRenderer>().sprite = faces[faceIndex]; 5}
FunctionRandom.Range(a, b)
randomizes between minimum value and maximum (non inclusive) - in this case a number of provided dice sprites
Testing the dice Roll
Every time you click the button, the dice will now roll a random number between one and six, updating the sprite to match the rolled number.
That's it! You can use this mechanic in your RPG games and much more. Have fun creating your random dice roll feature!