Create Stopwatch and Timer in Unity
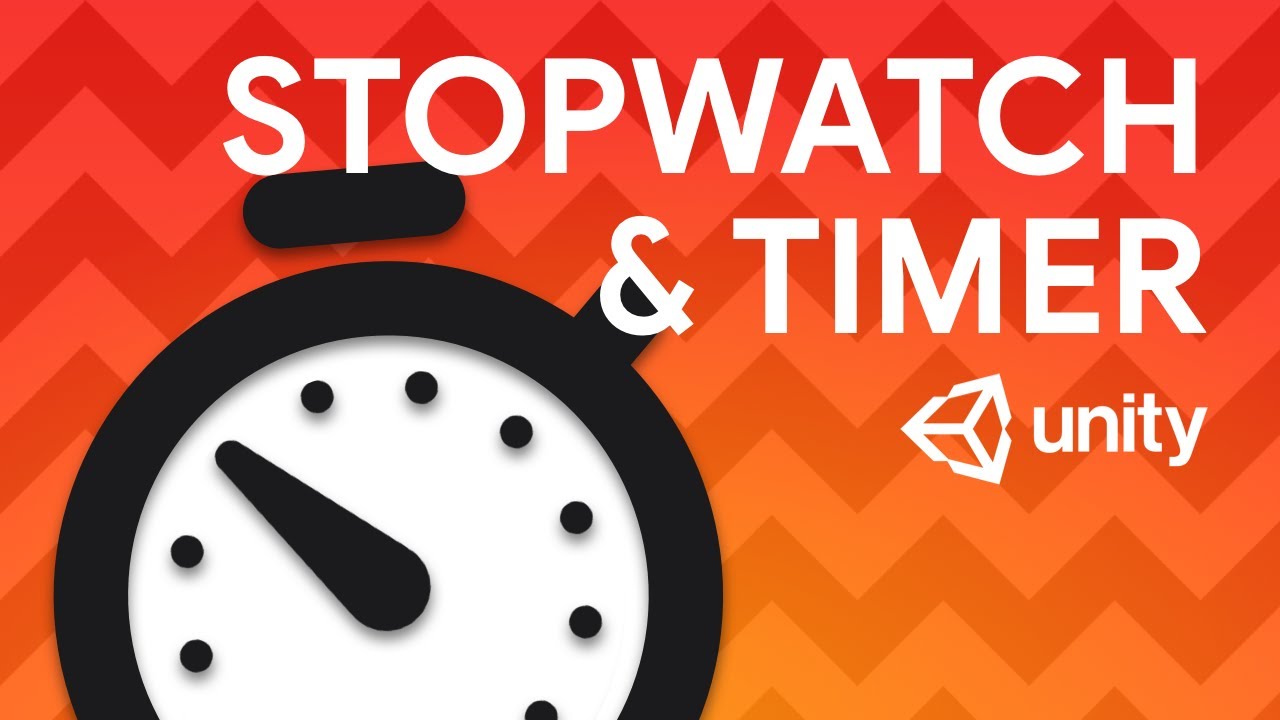
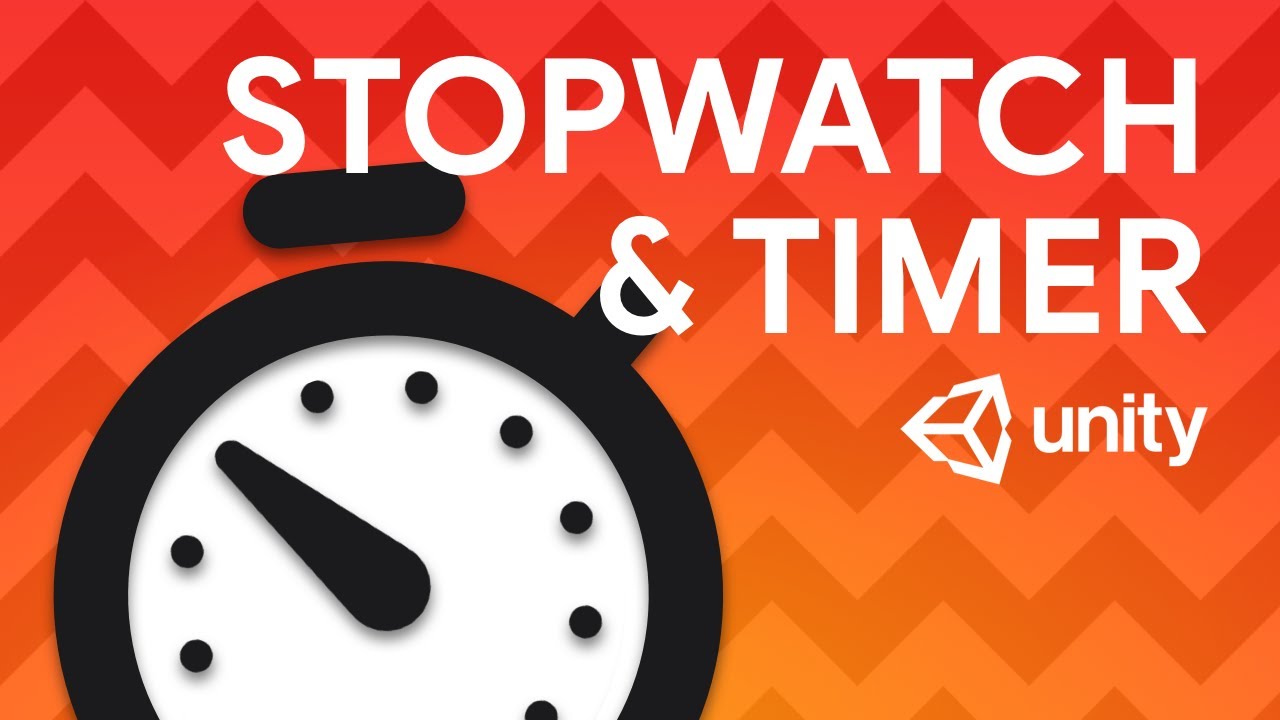
Create TIMER and STOPWATCH in your Unity game!
The most versatile thing you can add to your project is TIMER! It’s a core feature used in countless games like:
- Racing Games
- Endless Runners
- Survival modes
Today you will not only learn how to create countdown timer but also a stopwatch and how to calculate score in your game based on the time passed
Set up UI for the timer!
- Add text (TextMeshPro) to your scene showing “0:00” - this will be our timer
- Add two timer buttons one for the “Start” and the one for “Stop”
Preview
Create timer script
Lets’s create logic behind the countdown timer:
- Create new script called
Timer
:
C#1using UnityEngine;
2using UnityEngine.UI;
3
4public class Timer : MonoBehaviour {
5 public int StartMinutes;
6 public Text currentTimeText;
7
8 float currentTime;
9
10 void Start(){
11 currentTime = startMinutes * 60
12 }
13
14 void Update()
15 {
16 currentTimeText.text = currentTime.ToString();
17 }
18}
-
Add this script to any object on the screen, e.g. Canvas
-
Configure parameters:
- Start minutes: 0
- Current Time Text: drag in your text component
Preview -
To make time update every second, modify
Update
method. Let’s also addStartTimer
andStopTimer
:C#1void Update() 2{ 3 if (timerActive == true) 4 { 5 CurrentTime = currentTime - Time.deltaTime; 6 } 7 currentTimeText.text = currentTime.ToString(); 8} 9 10public void StartTimer() 11{ 12 timerActive = true; 13} 14 15public void StopTimer() 16{ 17 timerActive = false; 18}
-
Save script. Now in Unity go to “Start timer” button and in
OnClick
event assignTimer
script and selectTimer > StartTimer
method.Preview -
Repeat the same for Stop timer button
Great! Now our Timer runs when we click “Start”!
Preview
Time is not formatting properly?
If you start your timer now you will see that time is only measured in the seconds. To fix that
you need to use this simple method:
-
In your Update method, add this lineC#
1void Update() 2{ 3 TimeSpan time = TimeSpan.FromSeconds(currentTime); 4 currentTimeText.text = time.Minutes.ToString() + ":" + time.Seconds.ToString(); 5}
But if the time runs out then… oh no it goes negative!!!
-
You need to add new if statement to fix that. Here is a fully finished Update functionC#
1void Update() 2{ 3 if (timerActive == true) 4 { 5 currentTime = currentTime - Time.deltaTime; 6 if (currentTime <= 0) 7 { 8 timerActive = false; 9 Start(); 10 Debug.Log("Timer finished!"); 11 } 12 } 13 14 TimeSpan time = TimeSpan.FromSeconds(currentTime); 15 currentTimeText.text = time.Minutes.ToString() + ":" + time.Seconds.ToString(); 16}
Let’s create stopwatch!
If you created timer now its time for stopwatch (I promise its only getting easier from here)
- Copy the scene from Timer
- Duplicate timer script
- Rename all the files to mention the stopwatch (or just copy script below)
To make Stopwatch work:
- Set the start time to 0
- Alter update function so that
currentTime
will be increased overtime:
C#1using UnityEngine;
2using UnityEngine.UI;
3using System;
4
5public class Stopwatch : MonoBehaviour
6{
7 bool stopwatchActive = false;
8 float currentTime;
9 public Text currentTimeText;
10
11 void Start()
12 {
13 currentTime = 0;
14 }
15
16 void Update()
17 {
18 if (stopwatchActive == true)
19 {
20 currentTime = currentTime + Time.deltaTime;
21 }
22
23 TimeSpan time = TimeSpan.FromSeconds(currentTime);
24 currentTimeText.text = time.Minutes.ToString("00") + ":" + time.Seconds.ToString("00");
25 }
26
27 public void StartStopwatch()
28 {
29 stopwatchActive = true;
30 }
31
32 public void StopStopwatch()
33 {
34 stopwatchActive = false;
35 }
36
37 public void ResetStopwatch()
38 {
39 currentTime = 0;
40 currentTimeText.text = "00:00";
41 }
42}
-
To add millisecond to your stopwatch (For you speed runners 😉), use this line:C#
currentTimeText.text = time.ToString(@"mm\:ss\:fff");
-
Just like that we created timer!
Add a score system based on time!
So whats the time if you cant set new high scores right? Now let’s create score measurement system perfect for runner games
-
Duplicate your stopwatch script
-
Add
scoreText
text -
Your new gameManager:C#
1using UnityEngine; 2using UnityEngine.UI; 3using System; 4 5public class GameManager : MonoBehaviour 6{ 7 // Stopwatch 8 bool stopwatchActive = false; 9 float currentTime; 10 public Text currentTimeText; 11 12 // Score 13 int score; 14 public Text scoreText; 15 public float multiplier = 5; 16 17 void Start() 18 { 19 currentTime = 0; 20 score = 0; 21 scoreText.text = score.ToString(); // Initialize score text 22 } 23 24 void Update() 25 { 26 if (stopwatchActive == true) 27 { 28 currentTime = currentTime + Time.deltaTime; 29 30 // Score Part 2 31 score = Mathf.RoundToInt(currentTime * multiplier); 32 scoreText.text = score.ToString(); 33 } 34 35 TimeSpan time = TimeSpan.FromSeconds(currentTime); 36 currentTimeText.text = time.ToString(@"mm\:ss\:fff"); 37 } 38 39 public void StartStopwatch() 40 { 41 stopwatchActive = true; 42 } 43 44 public void StopStopwatch() 45 { 46 stopwatchActive = false; 47 } 48 49 public void ResetStopwatchAndScore() 50 { 51 currentTime = 0; 52 stopwatchActive = false; 53 score = 0; 54 currentTimeText.text = "00:00:000"; // Reset time text 55 scoreText.text = score.ToString(); // Reset score text 56 } 57} 58
-
Drag text to current variable field
-
And that's it!
Now you’ve got working timer, stopwatch and score counter ready to implement to your games!
Hi! Why don’t you visit my discord?
Copyright © 2024-2025 Teal Fire