Load files from Assets folder using code (in Unity)
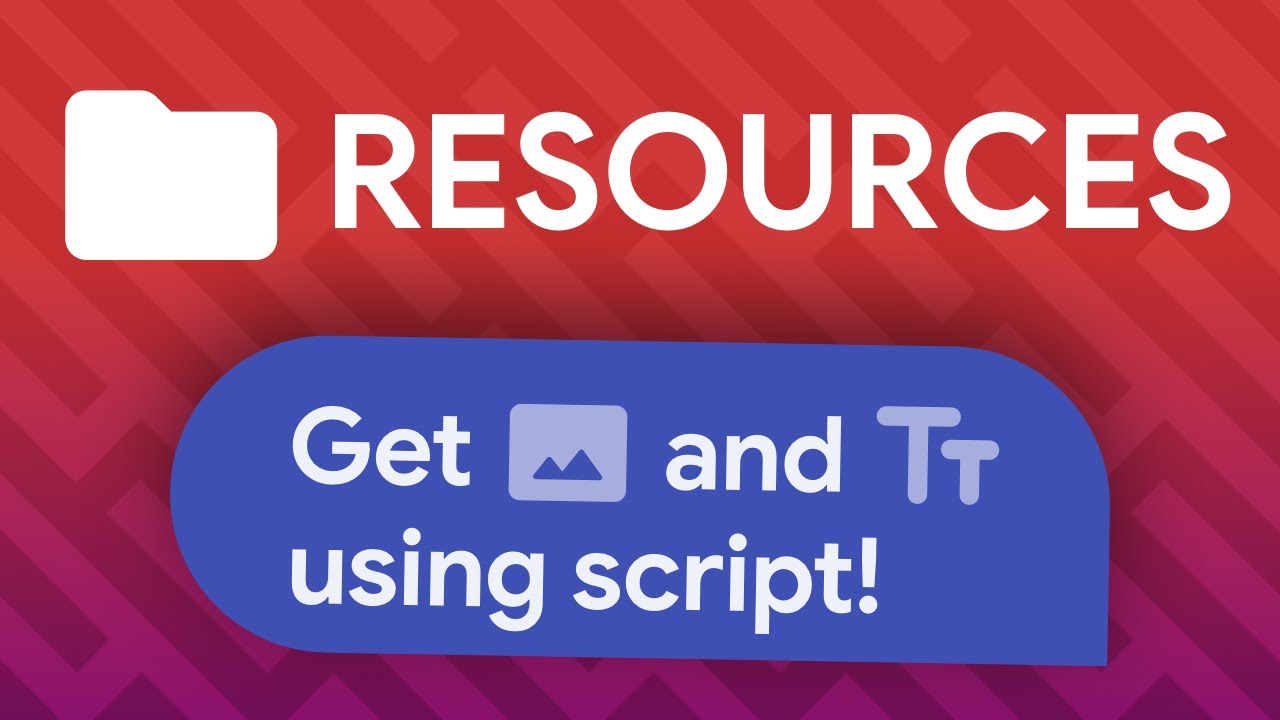
Loading...
Managing your assets can be straightforward when you are dealing with a few items: Simply drag your image from the asset folder into the corresponding field, and you are good to go!
But what if you have over 100 fields or other dynamic values! Dragging each one individually can be time consuming and not effective. Luckily, you can automate this process by importing them directly from the Assets folder using a script!
Setting up your assets folder
First, you’ll need to create a specific folder in your project
- Create new folder called Resources (name is very important!)
- Inside of it add a new folder, for example “Icons”
- Drag all of your assets in to Resources/Icons folder. In this example just import some images.
Writing the script to load assets
Now that you have your assets organised, it is time to write some code to load them. We can load assets individually using path, but here is how to load all the assets in one folder
-
Create a new script called
LoadAssets
. Inside, in the Start function type:C#1void Start() { 2 sprites = Resources.LoadAll("Icons", typeof(sprite)) 3}
Replace “Icons” with your real name of the folder inside the Resources :) -
LoadAll
returns an array of generic objects. Here is how to cast them to the correct type:C#sprites = Resources.LoadAll("Name of your folder", typeof(sprite)).Cast<Sprite>().ToArray();
-
You may need to manually add this line at the top if you see errors:C#
using system.Linq;
-
Make use of this variable - for example you can
Instantiate
new objects on the scene.
Run your game: After saving your script, return to your scene and run your game. If you did everything correctly you will see that sprites are loaded as planned!
/image.png)
Preview
Loading text files
Loading texts files to your games is very simple and similar process. Here is how to do it:
-
Go back to your script and add the following line:C#
1void Start() { 2 TextAsset message = (TextAsset)Resources.Load("NameOfYourTextFile"); 3}
Replace Name Of Your Text File with your real name of text file :) -
Assign string to text componentC#
1public TMP_Text header; 2 3void Start() { 4 TextAsset message = (TextAsset)Resources.Load("NameOfYourTextFile"); 5 header.text = message.text; 6}
-
Test it Out: Save your script and run your game. You’ll see that text is loaded up and is displayed as expected. If you update your text file and restart scene, the text in game will also update accordingly.
And that's it! You've successfully learned how to dynamically load assets and text files in Unity.
This method is a fantastic way to keep your project organised. If you want more tutorials check out:
Hi! Why don’t you visit my discord?
Copyright © 2024-2025 Teal Fire